SQL Advanced 2: Temp Tables
SQL
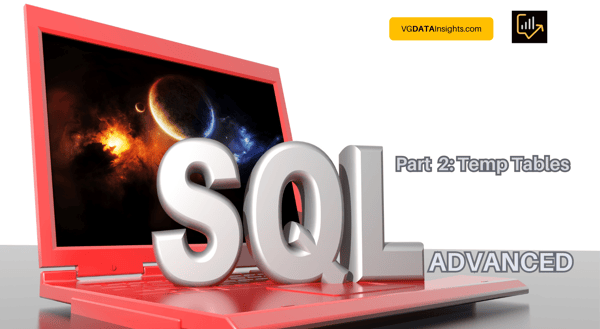
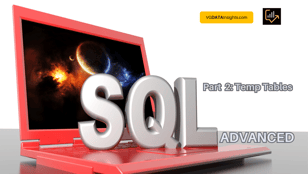
Join us for the second installment of our SQL Advanced series as we delve into the world of temporary tables. In this beginner-friendly post, we'll demystify the concept of temporary tables in SQL, exploring their benefits, use cases, and practical application in query optimization and data manipulation. Whether you're a newcomer to SQL or an experienced user looking to enhance your skills, this guide will equip you with the essential knowledge to make the most of temporary tables in your database operations.
Hello everyone and welcome to our second SQL Advanced series post about Temporary Tables. That is a lot to be said about temporary tables, however today, let’s have a beginner overview of it.
When it comes to managing and manipulating data in a relational database, SQL (Structured Query Language) is a powerful tool. One concept that might seem confusing at first but is incredibly useful is the concept of temporary tables. In this beginner's guide, we'll dive into what temporary tables are, why they are useful, and how to use them effectively in your SQL queries.
What Are Temporary Tables in SQL?
Temporary tables, as the name suggests, are tables that exist temporarily within a database session. They are created to store and manipulate intermediate results or data that we don't need to store permanently.
Temporary tables are particularly handy when we need to break down complex queries into simpler steps, reuse subsets of data, or perform multiple operations on the same data without affecting the main database tables.
Why Use Temporary Tables in SQL?
Temporary tables offer several advantages:
Isolation: Temporary tables are only accessible within the session that created them. This isolation ensures that different users or sessions can use temporary tables without interfering with each other.
Performance: By storing intermediate results in temporary tables, we can optimize complex queries. Instead of repeatedly calculating the same values, we can store them once and reference them as needed.
Breaking Down Complex Queries: Large, complex queries can be challenging to manage and optimize. Temporary tables allow us to break down a complex task into smaller, more manageable steps.
Reuse of Data: If a subset of data is required for multiple operations, we can store it in a temporary table and reuse it without having to re-query the main tables.
Debugging: When troubleshooting complex queries, temporary tables can help by allowing us to inspect intermediate results at various stages of the query.
Creating Temporary Tables in SQL
Temporary tables are typically created in one of two ways:
Local Temporary Tables: These are session-specific and are only visible to the session that created them. They are defined with a single hash (#) sign before the table name. Once the session ends, the table is automatically dropped.
If you like this content and want to learn more, you might also read:
SQL Basics 1: How to Create Table and Add Data in SSMS
SQL Basics 2: SELECT + FROM Statements
SQL Basics 4: GROUP BY and ORDER BY Statements
SQL Intermediate 1: INNER and OUTER Joins
SQL Intermediate 2: UNION Operator:
SQL Intermediate 3: CASE Statement
SQL Intermediate 4: HAVING Clause
SQL Intermediate 5: UPDATE and DELETE Data
SQL Intermediate 6: AS - Aliasing
SQL Intermediate 7: PARTITION BY Clause
SQL Advanced 1: CTEs - Common Table Expressions
SQL Advanced 3: Stored Procedures
SQL Advanced 4: SUBQUERIES - In Select, From and Where
SQL Advanced 5: String Functions - TRIM, LTRIM, RTRIM, REPLACE, SUBSTRING, UPPER, and LOWER
Global Temporary Tables: These are visible to all sessions within a database but are deleted when the last session referencing them is closed. They are defined with two hash (##) signs before the table name.
Using Temp Tables in SQL:
Once we've created a temporary table, we can manipulate and query it just like any other table:
-- Insert data into the temporary table:
INSERT INTO #TempTable (ID, Name) VALUES (1, 'Alice'), (2, 'Bob');
-- Query data from the temporary table
SELECT * FROM #TempTable;
-- Update data in the temporary table
UPDATE #TempTable SET Name = 'Charlie' WHERE ID = 2
-- Delete data from the temporary table
DELETE FROM #TempTable WHERE ID = 1
Maximizing Temporary Table Usage in SQL:
We do not necessarily need to insert data into a Temp Table, we can also select all data from a specific table or a subset of it and insert into there.
For example, let’s create a Temp Table with values EmployeeID, JobTitle and Salary, similar to our ‘EmployeeSalary’ table to paste its data into the Temp Table.
CREATE TABLE #Temp_Employee
(EmployeeID int,
JobTitle varchar(50),
Salary int)
INSERT INTO #Temp_Employee
SELECT *
FROM EmployeeSalary
SELECT *
FROM #Temp_Employee
We moved information from the 'EmployeeSalary' table to our own '#Temp_Employee' table. This is handy when we want to work with complicated queries that involve combining data from different sources, like using joins or special functions. With a temp table, we can focus on a smaller part of the data that we need for all the upcoming queries.
Another more advanced example is if we want to create a temp table to store aggregated information about job titles, including the number of employees, average age, and average salary associated with each job title.
CREATE TABLE #Temp_Employee2
(JobTitle varchar(50),
EmployeesPerJob int,
AvgAge int,
AvgSalary int)
INSERT INTO #Temp_Employee2
SELECT JobTitle, COUNT(JobTitle), AVG(Age), AVG(Salary)
FROM EmployeeDemographics AS Demo
JOIN EmployeeSalary AS Sal
ON Demo.EmployeeID = Sal.EmployeeID
GROUP BY JobTitle
SELECT *
FROM #Temp_Employee2
After creating the ‘#Temp_Employee2’ table, the given code consists of two SQL statements:
INSERT INTO #Temp_Employee2: This statement populates the temporary table "#Temp_Employee2" with aggregated data. It performs the following actions:
It selects data from two source tables: "EmployeeDemographics" and "EmployeeSalary", which are joined using the common column "EmployeeID".
It calculates aggregated values for each "JobTitle":
The COUNT(JobTitle) calculates the number of employees for each job title.
The AVG(Age) calculates the average age of employees for each job title.
The AVG(Salary) calculates the average salary of employees for each job title.
The results are grouped by the "JobTitle" column.
SELECT * FROM #Temp_Employee2: This statement retrieves all the rows and columns from the temporary table "#Temp_Employee2" that was populated in the previous step. It effectively displays the aggregated data in tabular form.
Now that we have created ‘#Temp_Employee2’ table, instead of joining ‘EmployeeDemographics’ and ‘EmployeeSalary’ Table, then doings these calculations every time for further analysis, we can just use the temp table. It will dramatically reduce our runtime.
Temp Tables in Stored Procedures SQL:
Temporary tables are a valuable resource in stored procedures for managing and manipulating data during the execution of a sequence of SQL statements. When a stored procedure is executed, it might generate an output that needs to be processed further or used for multiple iterations. However, using temporary tables within stored procedures can sometimes lead to challenges such as encountering errors due to table name conflicts.
For example, when executing a stored procedure multiple times, a common issue arises where the temporary table used in the procedure already exists from a previous run, resulting in an error message like:
To address this issue and ensure smooth execution, a helpful strategy involves incorporating the:
DROP TABLE IF EXISTS statement.
This statement checks for the existence of the temporary table before attempting to drop it. If the table exists, it is dropped; if not, the statement simply continues without any errors.
Using the DROP TABLE IF EXISTS statement, as shown below, is a practical way to circumvent the error and maintain the flow of the stored procedure:
By implementing this approach, the stored procedure can be run multiple times without encountering table name conflicts, as the temporary table is properly managed by dropping it if it already exists. This practice ensures a smoother and more reliable execution process when temporary tables are employed within stored procedures.
Remember that temporary tables are only available within the scope of the session that created them. They won't interfere with permanent tables in our database, and we don't need to worry about explicitly dropping them when we are done; they will be cleaned up automatically.
Temporary tables in SQL provide a valuable tool for managing and optimizing complex queries, reusing data, and improving the overall performance of your database operations. By understanding how to create, use, and leverage temporary tables effectively, we'll be better equipped to tackle a wide range of data manipulation tasks. As we continue to explore SQL, keep temporary tables in your toolbox to help streamline our database operations and enhance your querying skills.